Starting with Graphics Programming can be daunting. I experienced this myself when I started learning the subject in 2021. But one thing is for certain, it definitely can be learned!
There are many amazing resources around. Some explain the basics, while others delve into the deep end with advanced rendering techniques. There are so many resources that it can be intimidating to find the right ones. Some are considered outdated, others can be too advanced for beginners.
That’s why I decided to write this post. To provide a subject-based list of resources aimed towards new or beginner-level graphics programmers. Either you can pick per subject what you want to investigate or use it as a roadmap for starting with graphics programming.
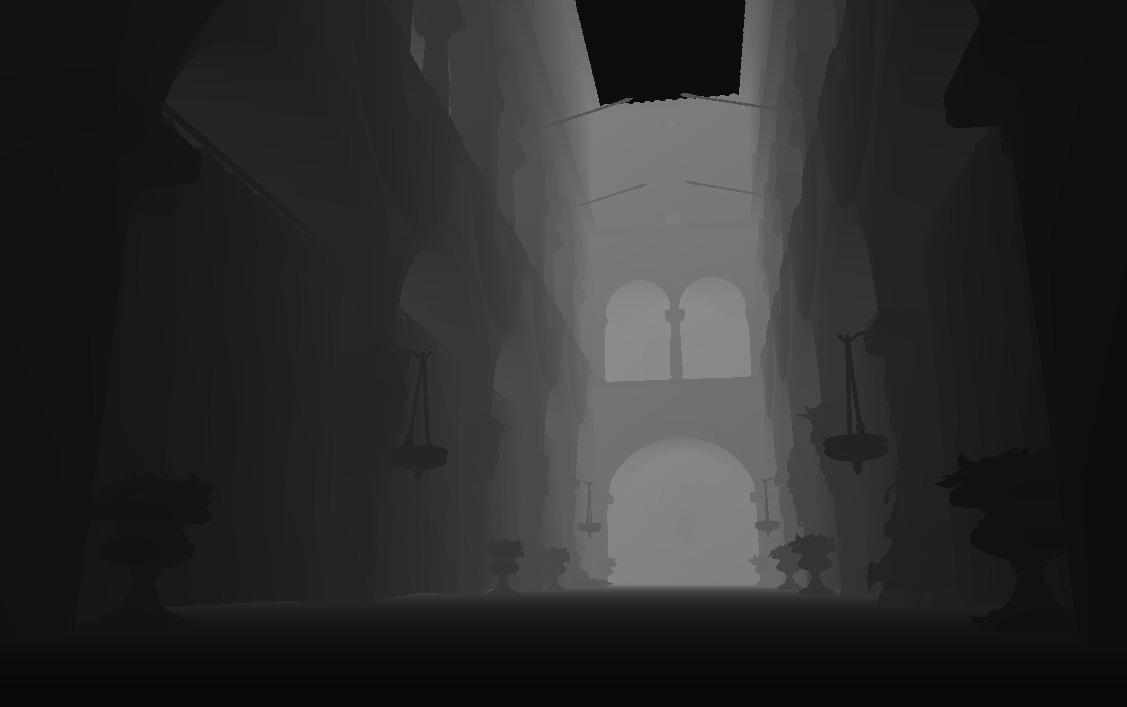
Heads up: Do note that I’m by no means a veteran with graphics, neither am I saying that this is “the way to learn graphics programming”. Instead, this is a compilation of resources that in my opinion were the most valuable to me when I was starting with graphics programming.
Subjects within Graphics Programming
Within graphics, there are a few important subjects to learn about if you wish to get a firm grasp on the foundations. Those topics are the following:
- Mathematics (Linear Algebra)
- Graphics API(s) & Rendering Techniques
- Shader Programming
You don’t need to have an expert-level understanding of all three topics to be a proficient graphics programmer. But, if you want to write renderers or create interesting shaders, a surface-level understanding of all these subjects will be required.
In practice, these subjects often overlap, and you can’t do one without the other. However, for the sake of learning more efficiently, it’s better to split them up first. For this reason, I wanted to provide individual resources so you can first familiarize yourself with the topics separately before combining them.
Prerequisites:
In general, it is assumed that when you look into this field you already have decent control of your preferred programming language.
You don’t have to be an expert! But a firm understanding of your language does allow you to understand the (pseudo-)code more quickly.
Do note that almost all resources use C++ for their examples. It’s still the preferred language for most resources surrounding graphics. But you don’t have to work in C++ to do graphics. Many languages like Python and Rust have compatible libraries for most APIs. So look around in case C++ isn’t your thing.
Learning Math for Graphics Programming
Whether you are a big fan of it or don’t understand it at all, mathematics is an essential part of graphics programming.
It allows us to transform all those triangles on the screen exactly how we want them to. Luckily, you don’t have to be a mathematician to start doing graphics programming. The topics within mathematics we need are quite standalone and don’t have many prerequisites to start learning.
To be able to start with graphics programming, a beginner/intermediate grasp of Linear Algebra is required. Specifically, you need to learn about vectors and matrices. These are fundamental concepts that not only allow us to move geometry (i.e. triangles, models) but also to make a renderer look 3D.
To learn these I recommend going to one or two of the following resources:
1. The Essence of Linear Algebra
This YouTube series by Mathematician Grant Sanderson, a.k.a. 3Blue1Brown is in my opinion the best introduction to Linear Algebra. It allows you to visualize what it means to use vectors and matrices. Which will help you understand what we do in graphics.
I recommend watching at least the first 5 videos. This gives you the basics to move on to the next resource(s). But feel free to continue watching. His playlist can be found here:
2. The 3D Math Primer
The ‘3D Math Primer for Graphics and Game Development’ by Fletcher Dunn and Ian Parberry is a comprehensive math book containing everything you need to know to fully start with Graphics. It starts simple and slowly builds up more and more concepts. It’s specifically aimed at people who are new to game engine/graphics mathematics.
You don’t need to go through the entire book to start with graphics, but I recommend reading the first 5-6 chapters while you work on your first renderer. Keep it close whenever you need an explanation for a topic.
The book can be bought on Amazon and similar sites, but is also available online for free here:
3. Foundations – Mathematics
The ‘Foundations of Game Engine Development’ book series by Eric Lengyel is a comprehensive collection of all sorts of subjects that relate to game engines. His first book in the series focuses solely on mathematics you can encounter within game engines.
I would recommend this book to people who feel quite confident with mathematics already and just want to know how math relates to the game engines/graphics. This book is less friendly towards people who are unfamiliar with math. For those people, I recommend the previous two resources instead.
More info about the book and where it can be bought can be found here:
Learning Graphics API(s) & Rendering Techniques
If you want to be able to write a renderer, then you will have to learn how to work with a Graphics API (e.g. OpenGL, DirectX). These APIs allow us to directly communicate with our GPU and execute our rendering techniques & shaders.
Starting with an API can be tricky since there are a lot of different things to learn. Luckily there are a few resources that are outstanding when it comes to learning the fundamentals. Chances are high you already landed on a few of them while looking into graphics programming.
These resources provide code, a clear explanation of how to set up & work with a given graphics API, and most explain many of the primary rendering techniques most renderers have. For this reason, I recommend starting with one of the following resources:
1. LearnOpenGL
Any graphics programmer who has started in the last 10 years has likely visited LearnOpenGL. This site written by Joey de Vries is one of the best starting points for any aspiring graphics programmer.
It gives a clear overview of rendering with OpenGL, and how a renderer works on a high level. It’s an excellent website with many topics to delve into in a chapter-based format. If you go through most of the content on the site chances are high you are ready to move on to more advanced topics & APIs.
LearnOpenGL is available online, in PDF format and even in physical book format. You can check it out right here:
2. OpenGL Dev
OGLDev by Etay Meiri is a well known tutorial series about modern OpenGL. OGLDev might be more interesting for you if you have preference for video tutorials.
Etay goes more in-depth about how certain rendering techniques work and explains them with animations in his videos. It helps you understand why rendering techniques work the way they do.
I would recommend his site and videos in case you want more detail about specific topics within graphics. But for a beginner, I wouldn’t recommend it as a series to follow from start to finish, use LearnOpenGL for that instead.
On his site you can find text format versions of his tutorials. On YouTube you can find some of his recent videos about graphics programming.
3. Learning DirectX 12
Learning DirectX 12 by Jeremiah van Oosten is a great tutorial for when you are completely new to the DirectX API. He explains step-by-step what you need to do to write a DirectX 12 renderer.
I recommend following this ONLY after making your first or second renderer with OpenGL. The tutorial doesn’t explain rendering techniques. It instead explains everything you need to know about the API since it assumes you already know the techniques when tackling DirectX 12.
Working towards learning DirectX 12 is a great thing to aspire to. It’s considered one of the industry-standard graphics APIs. Jeremiah’s tutorials about DirectX 12 can be found here:
Learning Shader Programming
With shaders we can run code on the GPU. Want your model to move? Then we need to transform our vertices in a vertex shader. Want your model to be red? Then we need to tell the fragment/pixel shader to output a red color.
Most tutorials mentioned above (e.g. LearnOpenGL) already go over shader programming since it’s a fundamental part of working with graphics APIs. So for this subject, I want to provide great resources that show you tricks and cool stuff you can do with shaders.
Having a good understanding of your tools within a shader will make it easier to implement rendering techniques. Knowing that, let’s go look at some resources to learn more about shaders on their own:
1. Book of Shaders
The Book of Shaders by Patricio Vivo and Jen Lowe is an interactive website where the basics of shader programming are explained.
The first three chapters explain your tools, what you can make with them and how to generate things like noise to make your shaders more interesting. It’s a nice introduction to what you can do within just a single shader.
At the moment of writing this, the site is still being worked on, so some chapters are unfinished. But the first few chapters are great so I highly recommend starting here. The site can be found here:
2. Kishimisu
Kishimisu has created two tutorials on Youtube that are in my opinion the best introductory tutorials for making Shader Art and doing Ray Marching.
The videos explain step-by-step different rendering techniques that can be used within shaders. It showcases that you can make wonderful creations with just a few lines of shader code.
Kishimisu’s YouTube channel with the tutorials can be found here:
3. ShaderToy
Now that you understand the fundamentals of shaders, it’s time to start making some of your own. Shadertoy by Inigo Quilez is a website where you can make and share your shaders.
The site is filled with submissions by many talented graphics/shader programmers. Some make realistic-looking worlds while others go for something stylized. All the shaders are public and you can learn a lot from dissecting them.
Making screen-space shaders like these is difficult at first, but it makes you understand what you can and cannot do. I highly recommend it. Go check out ShaderToy! Also, Inigo Quilez has a great site with articles about things you can use within your shaders:
The End
These were my suggested resources to start with graphics programming. Keep in mind that there are many others as well. But I can confidently say that if you follow some of the resources above then you are on your way to becoming a graphics programmer.
Thank you for checking out my (first) article!
I’m planning on doing more articles about Graphics in the future. If something like that is interesting to you make sure to follow me on my socials.
Cheers o/
Posted 23rd of March, 2024